- 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
public Class Singleton {
private static volatile Singleton instance;
private static Object lock=new Object();
private Singleton(){
}
public static Singleton getInstance(){
if(instance==null){
synchronized(lock){
instance=new Singleton();
}
}
}
return instance;
}
- 请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
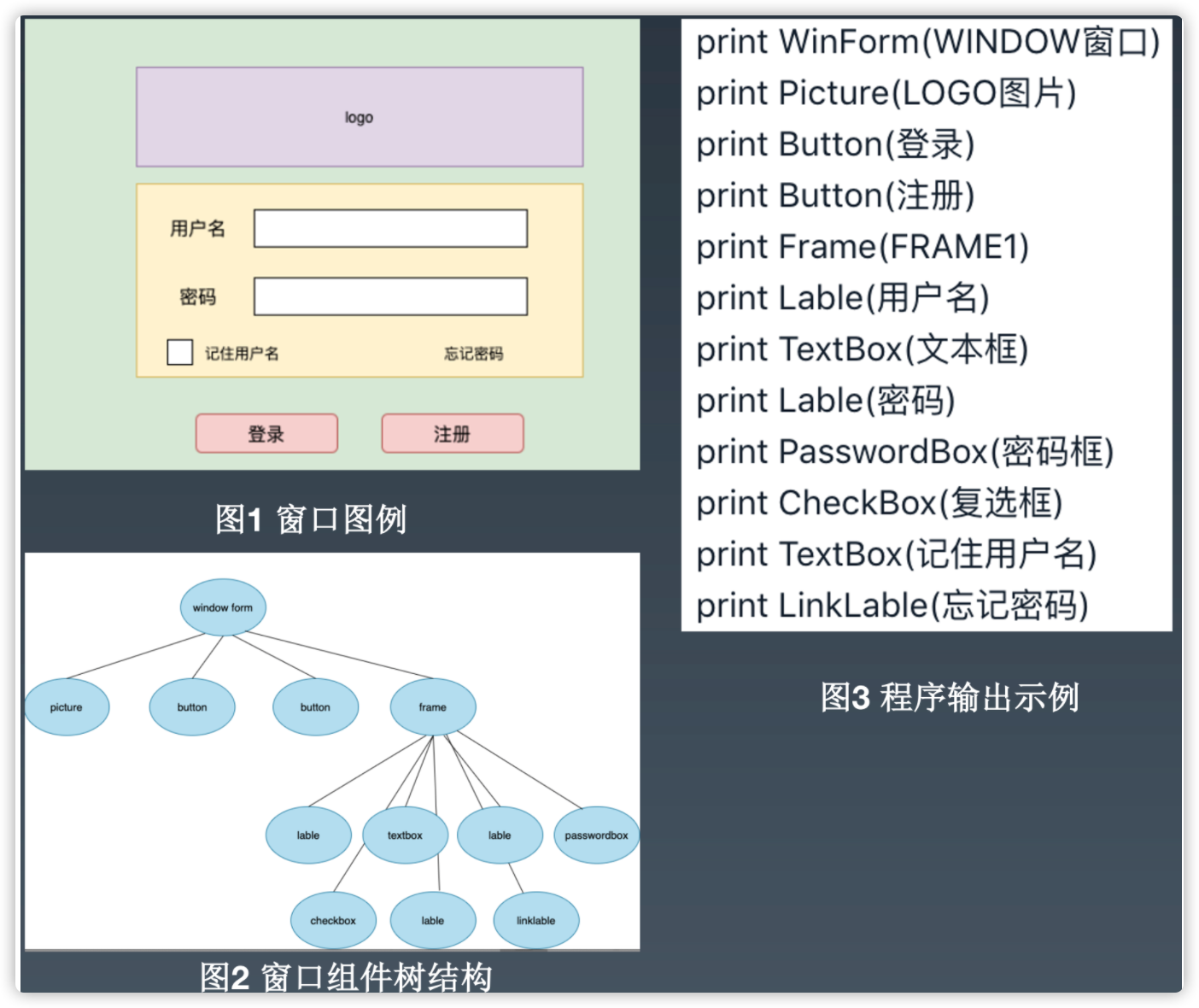
- 类设计图(设计的不太好,有很多优化点,比如类继承、容器的抽象)
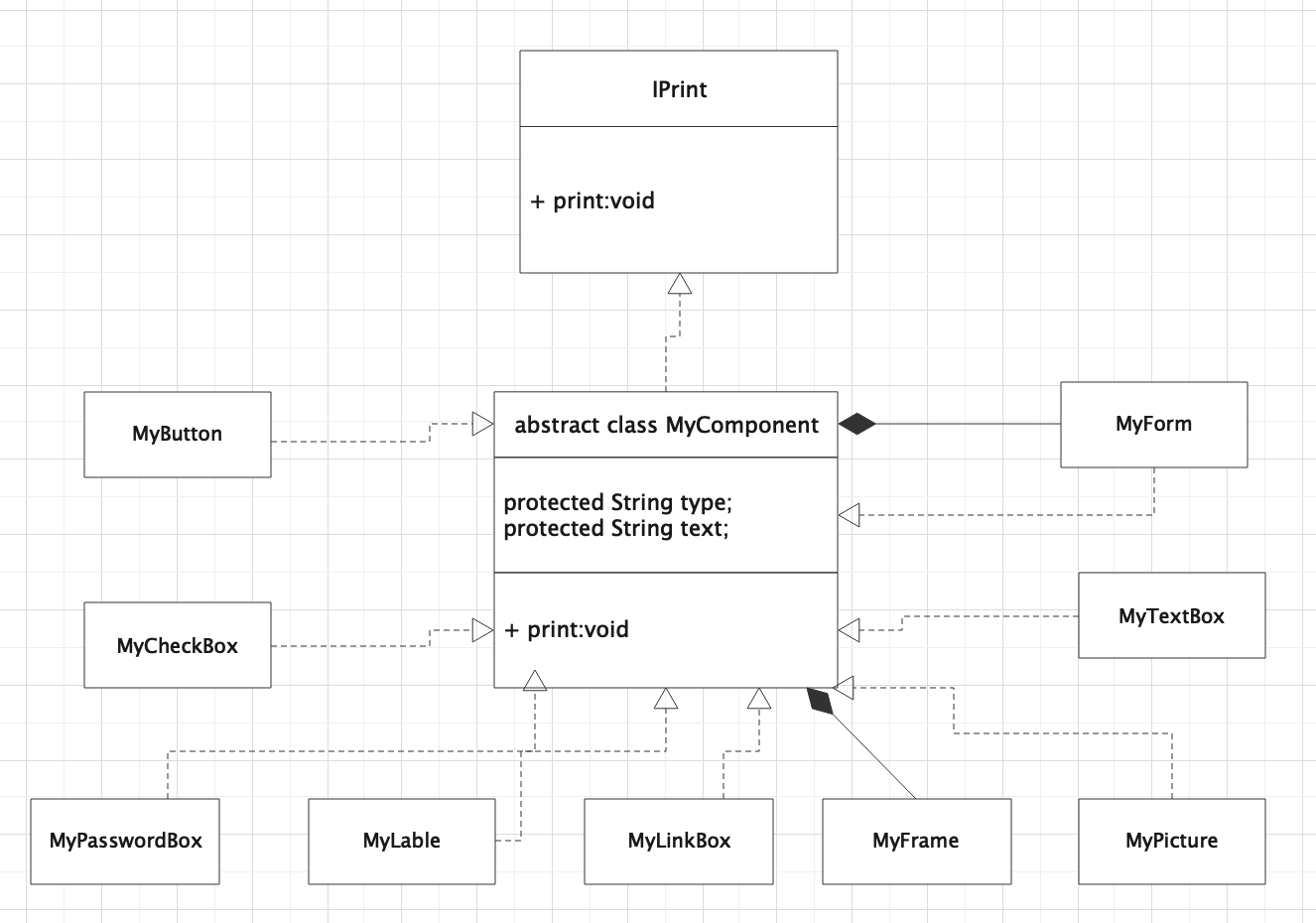
- 测试代码以及相关类
// 测试类
ublic class TestMain {
public static void main(String[] args) {
MyComponent form=new MyForm("WinForm");
MyComponent logo=new MyPicture("Logo");
MyComponent login=new MyButton("登录");
MyComponent reg=new MyButton("注册");
MyComponent userName=new MyLable("用户名");
MyComponent userPass=new MyLable("密码");
MyComponent userNameBox=new MyTextBox("用户名输入框");
MyComponent userPassBox=new MyPasswordBox("密码输入框");
MyComponent remember=new MyCheckBox("记住用户名");
MyComponent forget=new MyLinkBox("忘记密码");
MyComponent frame=new MyLinkBox("FRAME");
frame.append(userName);
frame.append(userPass);
frame.append(userNameBox);
frame.append(userPassBox);
frame.append(remember);
frame.append(forget);
form.append(logo);
form.append(login);
form.append(reg);
form.append(frame);
form.print();
}
}
public interface IPrint {
void print();
}
public abstract class MyComponent implements IPrint{
protected String type;
protected String text;
public MyComponent(String text){
this.text=text;
this.type=this.getClass().getSimpleName();
childs=new ArrayList<MyComponent>();
}
private List<MyComponent> childs;
public void append(MyComponent component){
this.childs.add(component);
}
public void print() {
System.out.println(this.type.substring(2)+"("+this.text+")");
for (MyComponent m:childs) {
m.print();
}
}
}
public class MyButton extends MyComponent {
public MyButton(String text) {
super(text);
}
}
public class MyCheckBox extends MyComponent {
public MyCheckBox(String text) {
super(text);
}
}
public class MyForm extends MyComponent {
public MyForm(String text) {
super(text);
}
}
public class MyFrame extends MyComponent {
public MyFrame(String text) {
super(text);
}
}
public class MyLable extends MyComponent {
public MyLable(String text) {
super(text);
}
}
public class MyLinkBox extends MyComponent {
public MyLinkBox(String text) {
super(text);
}
}
public class MyPasswordBox extends MyComponent {
public MyPasswordBox(String text) {
super(text);
}
}
public class MyPicture extends MyComponent {
public MyPicture(String text) {
super(text);
}
}
public class MyTextBox extends MyComponent {
public MyTextBox(String text) {
super(text);
}
}